Stripe
Using the provider
<?php
return [
'bnomei.kart.provider' => 'stripe',
// other options
];
Account
You need to create a Stripe account.
Test Mode and Secret Key
Ensure you are in Test Mode or a Sandbox and retrieve your Stripe Secret Key from the Stripe Dashboard.
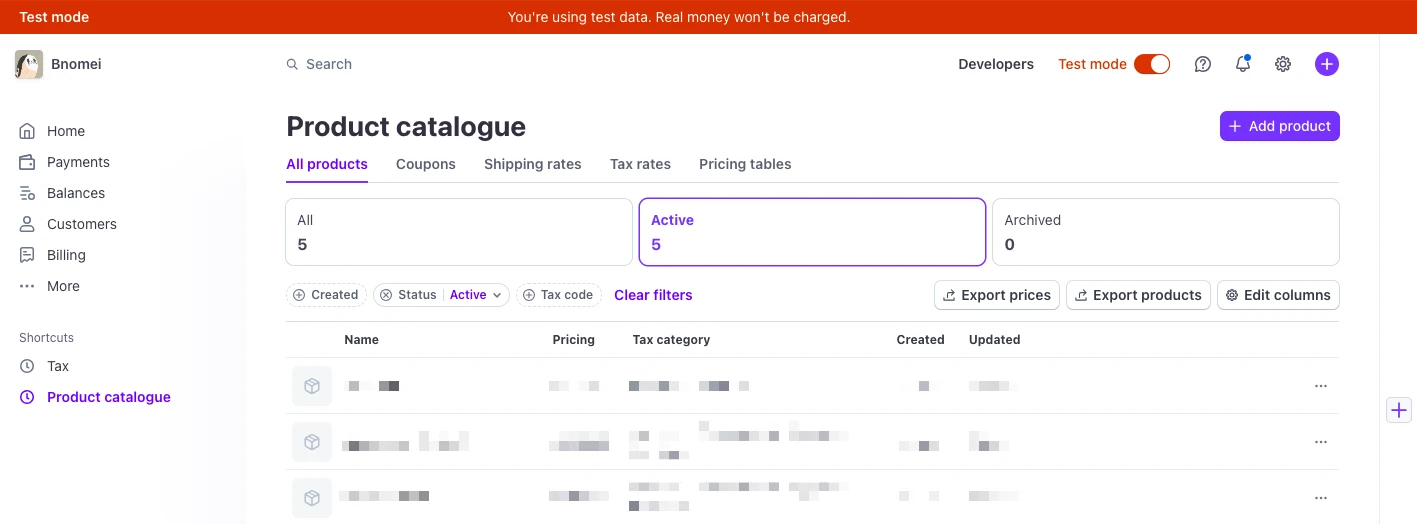
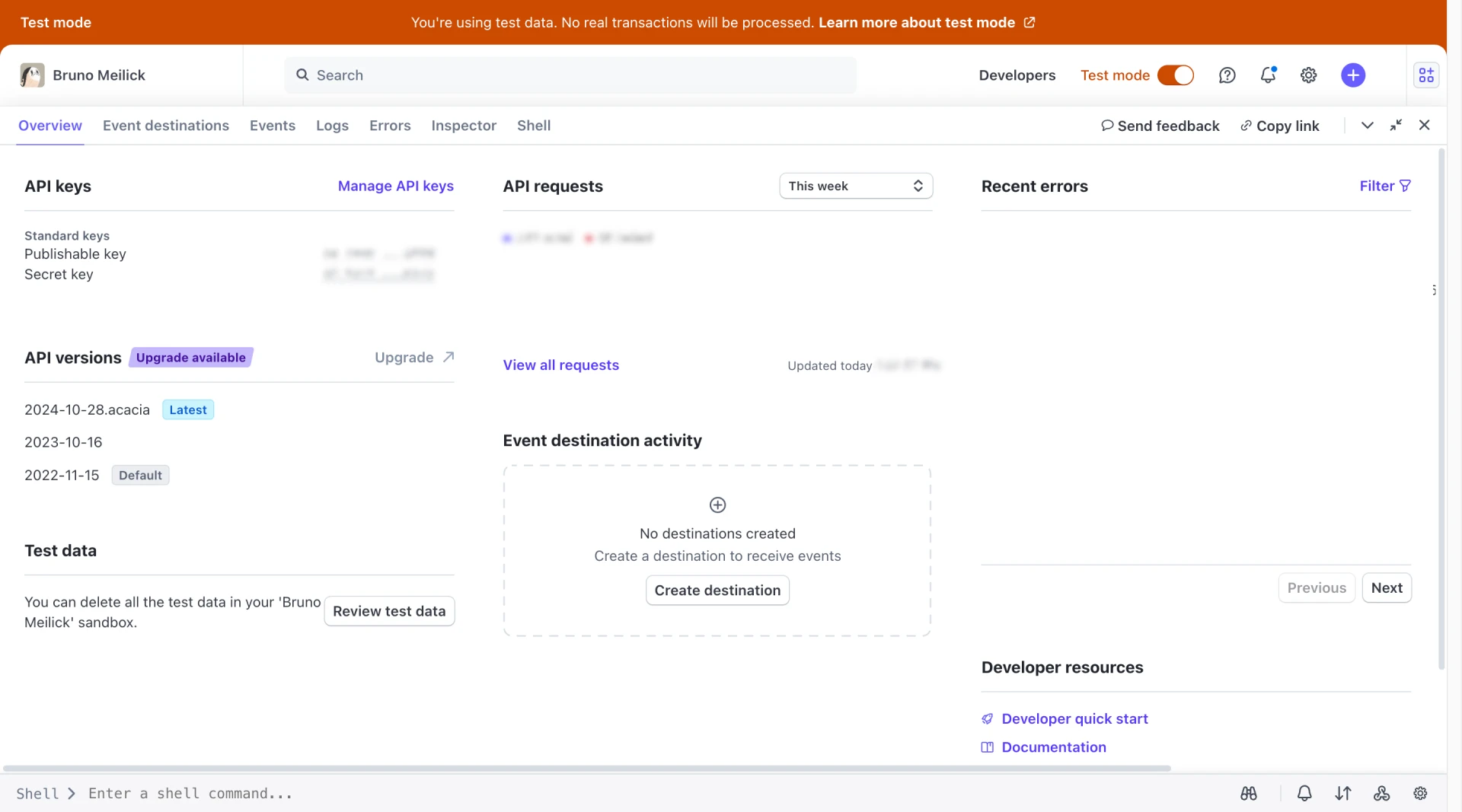
Configuration
Create a default configuration for the default with the Stripe STRIPE_SECRET_KEY_TEST
.
<?php
return [
'bnomei.kart.provider' => 'stripe',
'bnomei.kart.providers.stripe.secret' => fn() => env('STRIPE_SECRET_KEY_TEST'),
// other options
];
For your production domain I would recommend you create an additional configuration file and set the secret to either a different environment variable STRIPE_SECRET_KEY_LIVE
.
<?php
return [
'bnomei.kart.provider' => 'stripe',
'bnomei.kart.providers.stripe.secret' => fn() => env('STRIPE_SECRET_KEY_LIVE'),
// other options
];
You need to provide the credentials as config values as shown above or via an .env
file (plugin).
STRIPE_SECRET_KEY_TEST=XXX
STRIPE_SECRET_KEY_LIVE=ZZZ
Customzing the Checkout
You can use callbacks to customise both the checkout process and each line item. The returned arrays from your callbacks will be merged with the defaults created by the plugin for this provider, allowing you to override those defaults. Check the relevant PHP class and the linked online documentation for the provider to learn more about the expected data structure.
<?php
return [
'bnomei.kart.stripe.checkout_options' => function (\Bnomei\Kart\Kart $kart) {
// configure the checkout based on current kart instance
// https://docs.stripe.com/api/checkout/sessions/create
return [];
},
'bnomei.kart.stripe.checkout_line' => function (\Bnomei\Kart\Kart $kart, \Bnomei\Kart\CartLine $line) {
// add custom data to the current checkout line
return [];
},
// other options
];
API Version
You might want to react to the session data sent from Stripe at some point in your business logic. Within the Stripe Developer Dashboard you can set the API Version your account uses. Ensure you use the same version set in the dashboard when browsing their API docs for both the API and SDK.
The Kart plugin uses plain POST requests to the Stripe API and such is always the version you choose within the Stripe dashboard.
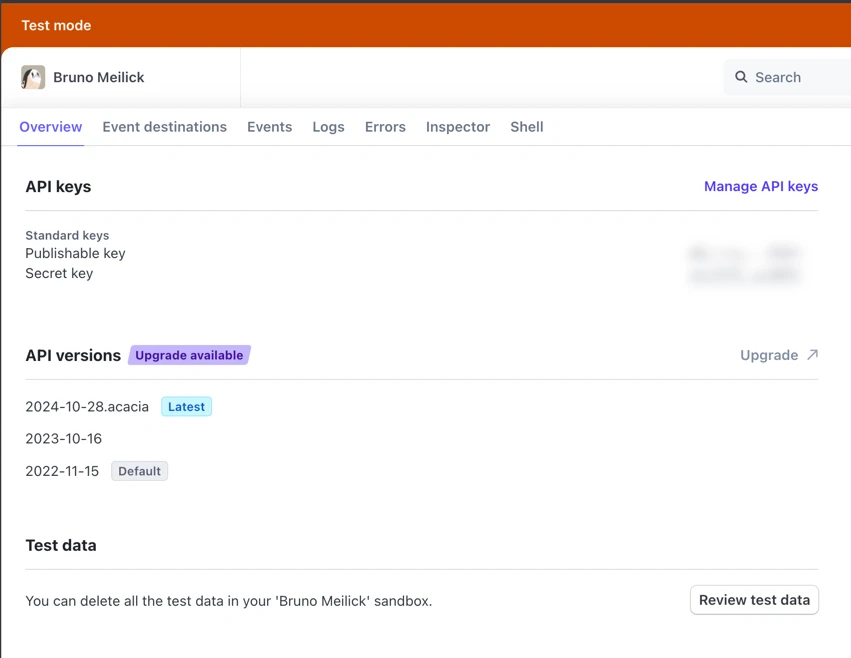
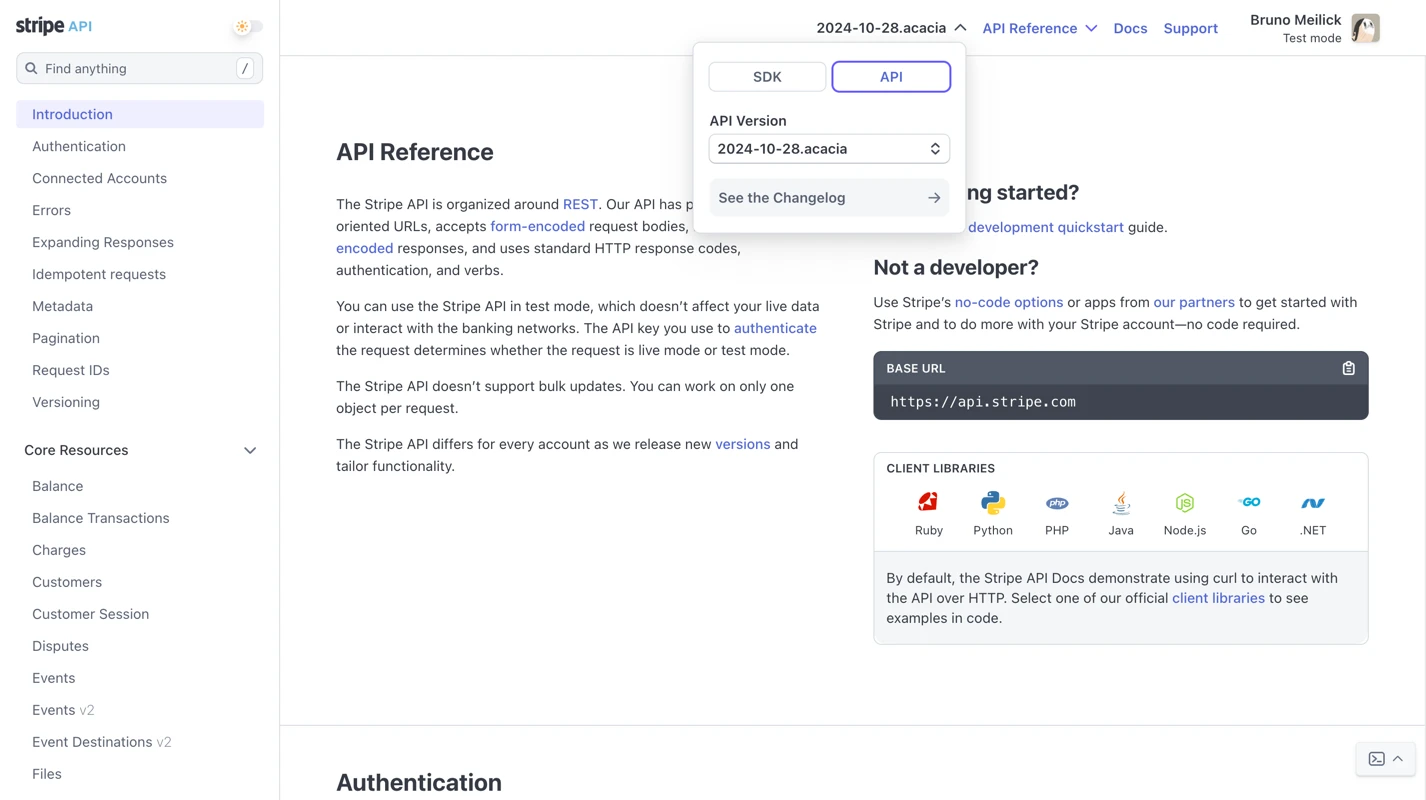
Customer Portal
You can link your customers to the provider portal with this code.
<a href="<?= kart()->provider()->portal() ?>">Stripe Portal</a>
Values from metadata
You can link categories, tags, gallery images, downloads, and the featured option from a product's metadata with a key to a value.
categories
=>evil
tags
=>light,red
gallery
=>filename_1.jpg,filename_2.jpg
downloads
=>nice-song.mp3
featured
=>true
Variants from prices
The Kirby Kart plugin will create a variant for each price. You can set custom metadata (in the price) with a key variant
, a comma-separated string of group:option
pairs, like size:M,color:Sky Blue
.